The Bitwise Shift Operators, as the name suggests, operate on the bit pattern by “shifting” it.
Bitwise Left Shift (<<)
The Left Shift Operator, as you can guess, shifts the bit pattern to the left.
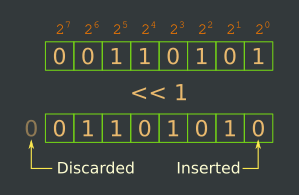
As the bits are shifted to the left, the right-most bit positions are filled with zeros.
Note that as the bits are shifted to the left, all the left-most bits shifted beyond the MSB position, are discarded.
Examples:
00110101 << 1 = 01101010 // Left shift by 1 position
10101101 << 1 = 01011010 // Left shift by 1 position
10101101 << 2 = 10110100 // Left shift by 2 positions
But, what is exactly happening here?
Let’s try to decipher.
Let's take the binary representation of 2 and left-shift it by 1 position.
0010 << 1 = 0100
Did you see what just happened?
0010 = 2
0100 = 4
Let's do it once more
0010 << 1 = 0100
0100 << 1 = 1000
In human language that means,
2 << 1 = 4
4 << 1 = 8
Do you see the pattern?
If we remember from the numbering systems article, the binary numbers are Base-2, which means that every position in the binary numbering system is 2 times the previous position.
Left-Shifting a binary digit by 1 position, essentially doubles it.
Let’s prove the above statement.
01010100 << 1 = 10101000
84 << 1 = 168 = 84 x 2
00110010 << 1 = 01100100
50 << 1 = 100 = 50 x 2
So, in fact, this also means that left-shifting a number by 2 positions will quadruple it.
00110010 << 2 = 11001000
50 << 2 = 200 = 50 x 4
And, so on and so forth...
Well, even if this is an interesting fact, and very handy at times, it does not have much real-life application. I mean, how many times do we need to multiply a number only by powers of 2 ( 2, 4, 8, 16, etc )? Surely not very often.
But, please hang-on, in the future articles, we will see the real-life application of these mystique operators. Till then trust me, bitwise operations do have real application!
Bitwise Right Shift (>>)
The Right-Shift operator has the same properties and characteristics as the Left-Shift operator, however, the bits are shifted to the Right instead.
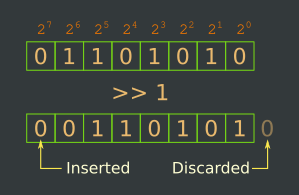
As the bits are shifted to the right, the left-most bit positions are filled with zeros.
Again, note that as the bits are shifted to the right, all the right-most bits shifted beyond the LSB position, are discarded.
Examples:
00110101 >> 1 = 00011010 // Right shift by 1 position
10101101 >> 1 = 01010110 // Right shift by 1 position
10101101 >> 2 = 00101011 // Right shift by 2 positions
Well, I am sure you know what I am going to say next!
If left-shift multiplies the number by powers of 2, then right-shift should divide the number by powers of 2. Right?!
Absolutely!! That’s exactly what happens!
Let’s prove
01010100 >> 1 = 00101010
84 >> 1 = 42 = 84 / 2
00110010 << 1 = 00011001
50 << 1 = 25 = 50 / 2
01100100 >> 2 = 00011001
100 >> 2 = 25 = 10 / 4
And, so on and so forth...